今回は入力データをPOST送信する方法をメモ
まずは、Formクラス。名前とメールを保持するクラス
package com.example.demo.form;
import lombok.Data;
@Data
public class Form {
private String name;
private String mail;
}
Controllerクラス
送信処理のsendメソッドの引数としてformオブジェクトを指定する必要があるのがポイント。
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import com.example.demo.form.Form;
@Controller
public class FormController {
/**
* 初期表示
* @param model
* @param form
* @return
*/
@GetMapping("/form")
public String form(Model model) {
return "form/index";
}
/**
* 送信完了画面
* @param model
* @param form
* @return
*/
@PostMapping("/send")
public String send(Model model, Form form) {
return "form/complete";
}
}
残りはView
th:action=”@{/send}”でサブミット先のアクション名を指定する。
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>お問い合わせフォーム</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
margin: 0;
padding: 0;
}
.container {
width: 500px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
}
h1 {
text-align: center;
margin-bottom: 20px;
}
form {
margin-bottom: 20px;
}
label {
display: block;
font-weight: bold;
margin-bottom: 10px;
}
input[type="text"] {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
input[type="submit"] {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
transition: background-color 0.3s;
}
input[type="submit"]:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<h1>お問い合わせフォーム</h1>
<form method="POST" th:action="@{/send}">
<div>
<label for="name">氏名:</label>
<input type="text" id="name" name="name" required>
</div>
<div>
<label for="mail">メールアドレス:</label>
<input type="text" id="mail" name="mail" required>
</div>
<div>
<input type="submit" value="送信">
</div>
</form>
</div>
</body>
</html>
最後にパラメータを受け取り表示するHTML
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>送信完了</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
margin: 0;
padding: 0;
}
.container {
width: 500px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
}
h1 {
text-align: center;
margin-bottom: 20px;
}
.info {
margin-bottom: 20px;
}
.info label {
font-weight: bold;
margin-bottom: 5px;
display: block;
}
.info div {
padding: 10px;
background-color: #f9f9f9;
border: 1px solid #ccc;
border-radius: 5px;
}
</style>
</head>
<body>
<div class="container">
<h1>送信完了</h1>
<div class="info">
<label>氏名:</label>
<div th:text="${form.name}"></div>
</div>
<div class="info">
<label>メールアドレス:</label>
<div th:text="${form.mail}"></div>
</div>
</div>
</body>
</html>
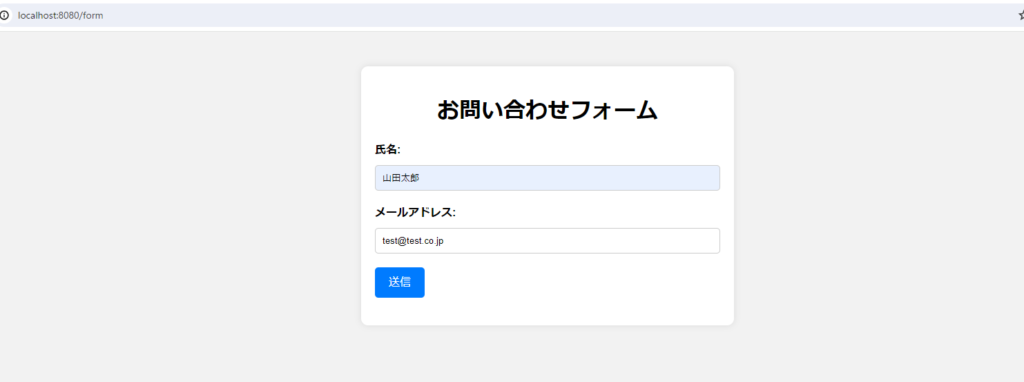
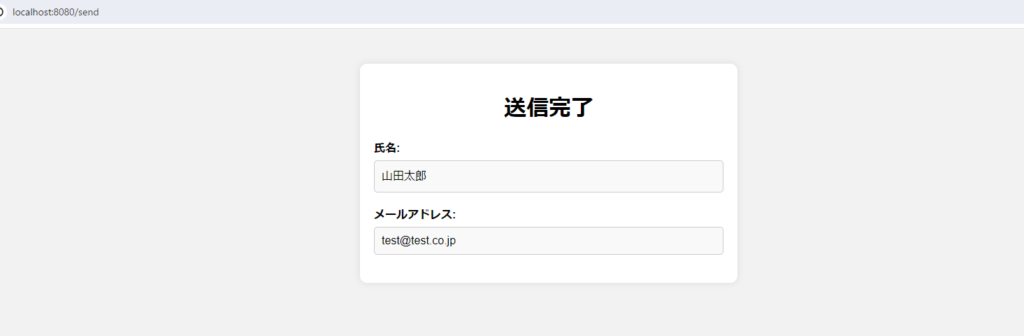
フォルダ構成
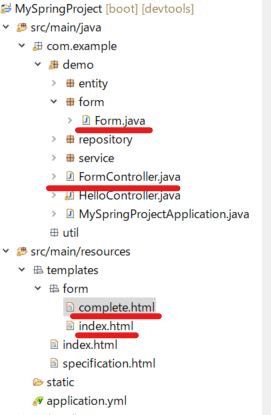