リダイレクト先にパラメータを渡す方法
初期画面で入力された名前を送信すると、リダイレクト先の画面に入力値が表示される。
コントローラクラス
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
@Controller
public class DemoController {
// 初期表示画面
@GetMapping("/")
public String index(Model model) {
// 初期画面へ
return "index";
}
// サブミット処理
@PostMapping("/submit")
public String submit(@RequestParam String username, RedirectAttributes redirectAttributes) {
// リダイレクト先へ値を設定
redirectAttributes.addFlashAttribute("username", username);
// 次の画面へリダイレクト
return "redirect:/next";
}
// サブミット画面からのリダイレクト先
@GetMapping("/next")
public String next(Model model) {
// リダイレクト時に渡されたユーザー名を取得
String username = (String) model.getAttribute("username");
// ビューにユーザー名を渡す
model.addAttribute("username", username);
// 次の画面へ
return "next";
}
}
リダイレクト先へデータを渡す場合は、
RedirectAttributesクラスの addFlashAttributeメソッドを使う
public String submit(@RequestParam String username, RedirectAttributes redirectAttributes) {
redirectAttributes.addFlashAttribute("username", username);
return "redirect:/next";
}
受け取る際は、ModelクラスのgetAttributeを使う
public String next(Model model) {
// リダイレクト時に渡されたユーザー名を取得
String username = (String) model.getAttribute("username");
入力画面のHTMLは以下の通り
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Hello world</title>
</head>
<body>
<form action="/submit" method="post">
名前:<input type="text" name="username">
<input type="submit" value="送信" />
</form>
</body>
</html>
リダイレクト先のHTML
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>次のページ</title>
</head>
<body>
受け取った値
<div th:text="${username}"></div>
</body>
</html>
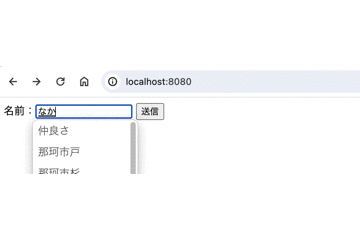