例えば以下のような関連テーブルがあるとする。
equipmentテーブル
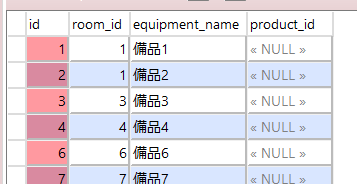
testテーブル
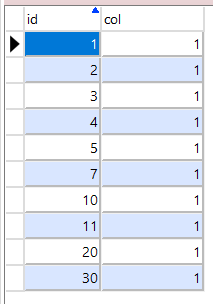
この2つのテーブルは1対多の関係にあるとする。
(equipmentのroom_idとtestのcolが紐づくとします)
※DB的には特に外部キーの設定はありません。
各テーブルのEntityを以下の通り定義する。
package com.example.demo.entity;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import org.hibernate.annotations.NamedQuery;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.Id;
import jakarta.persistence.OneToMany;
/**
* The persistent class for the equipment database table.
*
*/
@Entity
@NamedQuery(name="Equipment.findAll", query="SELECT e FROM Equipment e")
public class Equipment implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private int id;
@Column(name="equipment_name")
private String equipmentName;
@Column(name="room_id")
private int roomId;
@OneToMany(mappedBy = "equipment")
private List<Test> tests = new ArrayList<>();
public Equipment() {
}
// 以下省略
}
import java.io.Serializable;
import jakarta.persistence.Entity;
import jakarta.persistence.Id;
import jakarta.persistence.JoinColumn;
import jakarta.persistence.ManyToOne;
import jakarta.persistence.NamedQuery;
/**
* The persistent class for the test database table.
*
*/
@Entity
@NamedQuery(name="Test.findAll", query="SELECT t FROM Test t")
public class Test implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private int id;
// Equipmentとの関連を設定
@ManyToOne
@JoinColumn(name = "col") // Equipmentと結合するカラムを指定
private Equipment equipment;
public Test() {
}
public int getId() {
return this.id;
}
public void setId(int id) {
this.id = id;
}
}
親テーブル的には、@OneToManyのmappedBy プロパティに子テーブルのEntityで定義したプロパティ名を指定する
@OneToMany(mappedBy = "equipment")
private List<Test> tests = new ArrayList<>();
子テーブル的には@JoinColumnのname プロパティで親テーブルと紐づけたい子テーブルのカラム名を指定する。
// Equipmentとの関連を設定
@ManyToOne
@JoinColumn(name = "col") // Equipmentと結合するカラムを指定
private Equipment equipment;
実行時のSQL結果ログをを見ると以下の通り
2024-01-21T20:16:17.045+09:00 DEBUG 9748 --- [nio-8080-exec-1] org.hibernate.SQL :
select
e1_0.room_id,
e1_0.id,
e1_0.equipment_name
from
equipment e1_0
where
e1_0.room_id=?
2024-01-21T20:16:17.046+09:00 TRACE 9748 --- [nio-8080-exec-1] org.hibernate.orm.jdbc.bind : binding parameter (1:INTEGER) <- [1]
2024-01-21T20:16:32.852+09:00 DEBUG 9748 --- [nio-8080-exec-1] org.hibernate.SQL :
select
t1_0.col,
t1_0.id
from
test t1_0
where
t1_0.col=?
2024-01-21T20:16:32.852+09:00 TRACE 9748 --- [nio-8080-exec-1] org.hibernate.orm.jdbc.bind : binding parameter (1:INTEGER) <- [1]